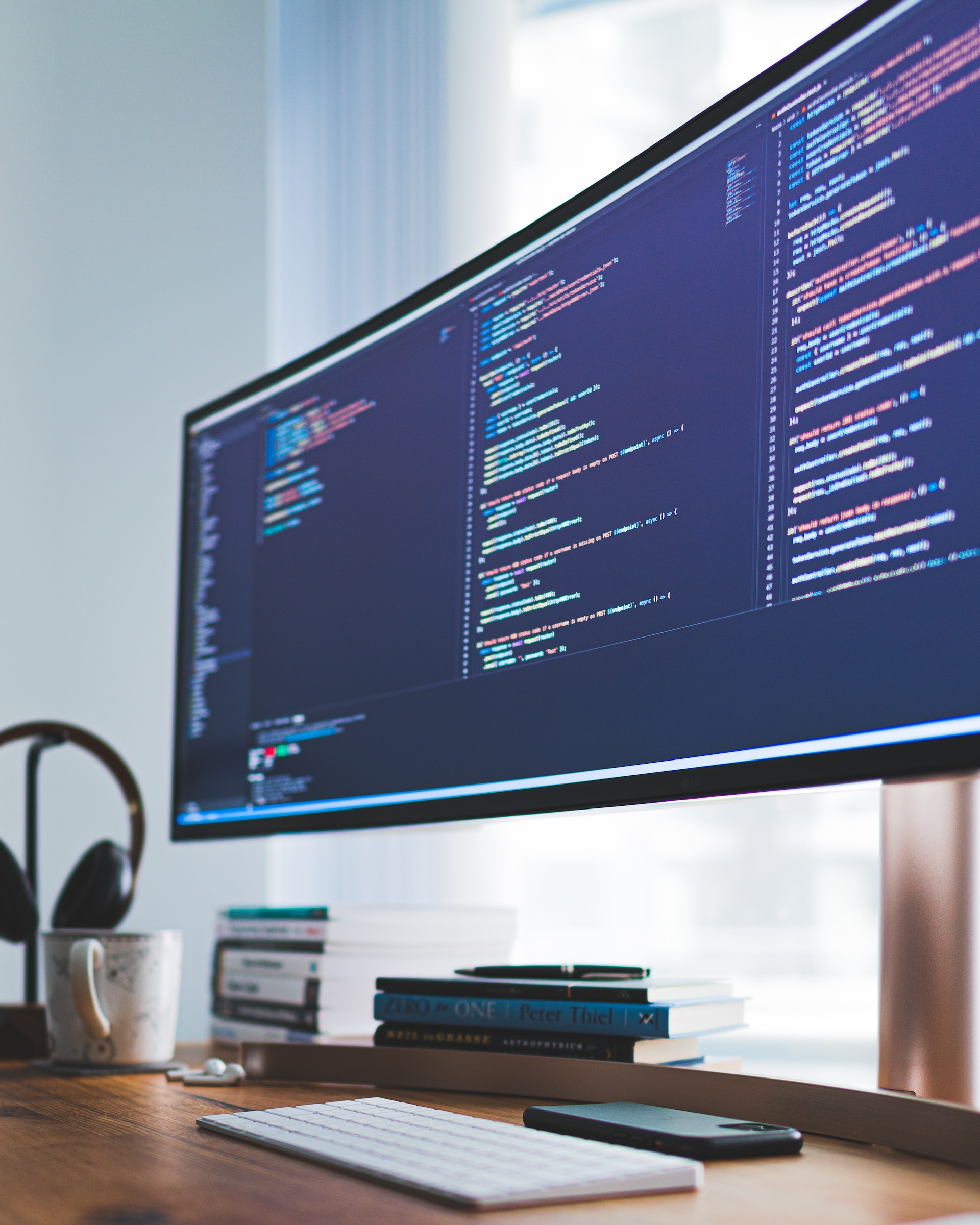
Exploring Component-Based Architectures: HTML Web Components vs React Components vs Angular Components
Creating a comprehensive comparison between HTML Web Components, React Components, and Angular Components involves various aspects such as syntax, features, and usage. Below is an article that provides an overview and includes a simple demo for each type of component.
Introduction:
In modern web development, component-based architectures have become a cornerstone for building scalable and maintainable applications. In this article, we'll explore three popular approaches to creating components: HTML Web Components, React Components, and Angular Components. Each approach has its strengths and use cases, and we'll compare them through practical examples.
1. HTML Web Components:
HTML Web Components are a set of web standards that enable the creation of reusable and encapsulated components. They consist of three main technologies:
- Custom Elements
- Shadow DOM, and
- HTML Templates.
Custom Elements:
Custom Elements allow developers to define their own HTML elements with their desired behavior. Let's create a simple custom element called "my-button."
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML Web Components Demo</title>
</head>
<body>
<my-button></my-button>
<script>
class MyButton extends HTMLElement {
connectedCallback() {
this.innerHTML = '<button>Click me</button>';
}
}
customElements.define('my-button', MyButton);
</script>
</body>
</html>
2. React Components:
React is a JavaScript library for building user interfaces, and its components are a fundamental part of the framework. Here's a simple React component that serves as a button:
import React from 'react';
class MyButton extends React.Component {
render() {
return <button>Click me</button>;
}
}
export default MyButton;
3. Angular Components:
Angular is a full-fledged framework that uses TypeScript to build web applications. Angular components are the building blocks of Angular applications. Below is an example of an Angular component:
import { Component } from '@angular/core';
@Component({
selector: 'app-my-button',
template: '<button>Click me</button>',
})
export class MyButtonComponent {}
Demo:
Now, let's compare the usage of these components in a simple web page.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Component Comparison Demo</title>
</head>
<body>
<!-- HTML Web Component -->
<my-button></my-button>
<!-- React Component -->
<div id="react-root"></div>
<script src="react.bundle.js"></script>
<script>
const element = React.createElement(MyButton);
ReactDOM.render(element, document.getElementById('react-root'));
</script>
<!-- Angular Component -->
<app-my-button></app-my-button>
<script src="angular.bundle.js"></script>
</body>
</html>
Comments